Vous devez être membre et vous identifier pour publier un article.
Les visiteurs peuvent toutefois commenter chaque article par une réponse.
Modifier le lazy loading avec Hibernate
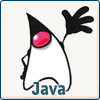
Généralités
Cet exemple fonctionne avec la version Hibernate 3.5.1-Final.
Il s’agit d’un aide mémoire pour moi, mais il peut servir à d’autres...
Procédure
RemoteLazyLoadCollectionCallback
- Dans org.hibernate.collection , ajouter le package callback
- Dans org.hibernate.collection.callback, ajouter la classe suivante :
Code Java (11 lignes)
- package org.hibernate.collection.callback;
- import org.hibernate.collection.PersistentCollection;
- import org.hibernate.engine.SessionImplementor;
- public interface RemoteLazyLoadCollectionCallback {
- PersistentCollection lazyLoad(SessionImplementor session,
- PersistentCollection collection);
- }
AbstractPersistentCollection
- Dans org.hibernate.collection, ouvrir la classe AbstractPersistentCollection.
-
Ajouter un membre statique :Code Java (9 lignes)
- private static RemoteLazyLoadCollectionCallback remoteLazyLoadCollectionCallback;
- /**
- * @param remoteLazyLoadCollectionCallback the remoteLazyLoadCollectionCallback to set
- */
- public static synchronized void setRemoteLazyLoadCollectionCallback(
- RemoteLazyLoadCollectionCallback remoteLazyLoadCollectionCallback) {
- AbstractPersistentCollection.remoteLazyLoadCollectionCallback = remoteLazyLoadCollectionCallback;
- }
- Ajouter un getter :
Code Java (3 lignes)
- private boolean isRemote() {
- return remoteLazyLoadCollectionCallback != null;
- }
- Dans la méthode protected boolean readSize(), ajouter au début
Code Java (3 lignes)
- if (isRemote()) {
- initialize(true);
- }
Faire de même pour toutes les méthodes readxxx. - Modifier la méthode protected final void initialize(boolean writing) comme ceci :
Code Java (15 lignes)
- protected final void initialize(boolean writing) {
- if (!initialized) {
- if (isRemote()) {
- remoteLazyLoadCollectionCallback.lazyLoad(session, this);
- setInitialized();
- } else {
- if (initializing) {
- throw new LazyInitializationException(
- "illegal access to loading collection");
- }
- throwLazyInitializationExceptionIfNotConnected();
- session.initializeCollection(this, writing);
- }
- }
- }
RemoteLazyLoadProxyCallback
- Dans org.hibernate.proxy , ajouter le package callback
- Dans org.hibernate.proxy.callback, ajouter la classe suivante :
Code Java (12 lignes)
- package org.hibernate.proxy.callback;
- import java.io.Serializable;
- import org.hibernate.engine.SessionImplementor;
- public interface RemoteLazyLoadProxyCallback {
- }
AbstractLazyInitializer
- Dans org.hibernate.proxy, ouvrir la classe AbstractLazyInitializer
- Ajouter un membre statique :
Code Java (14 lignes)
- private static RemoteLazyLoadProxyCallback remoteLazyLoadProxyCallback;
- /**
- * @param remoteLazyLoadProxyCallback the remoteLazyLoadProxyCallback to set
- */
- public static synchronized void setRemoteLazyLoadProxyCallback(
- RemoteLazyLoadProxyCallback remoteLazyLoadProxyCallback) {
- AbstractLazyInitializer.remoteLazyLoadProxyCallback = remoteLazyLoadProxyCallback;
- }
- Ajouter un getter :
- private boolean isRemote() {
- return (remoteLazyLoadProxyCallback != null);
- }
- Ajouter un getter :
Code Java (3 lignes)
- private boolean isRemote() {
- return (remoteLazyLoadProxyCallback != null);
- }
- Modifier la méthode public final void initialize() comme ceci :
Code Java (26 lignes)
- public final void initialize() throws HibernateException {
- if (!initialized) {
- if (isRemote()) {
- setImplementation(remoteLazyLoadProxyCallback.lazyLoad(session,
- entityName, id));
- } else {
- if ( session==null ) {
- throw new LazyInitializationException("could not initialize proxy - no Session");
- }
- else if ( !session.isOpen() ) {
- throw new LazyInitializationException("could not initialize proxy - the owning Session was closed");
- }
- else if ( !session.isConnected() ) {
- throw new LazyInitializationException("could not initialize proxy - the owning Session is disconnected");
- }
- else {
- target = session.immediateLoad(entityName, id);
- initialized = true;
- checkTargetState();
- }
- }
- }
- else {
- checkTargetState();
- }
- }
Exemple d’appel dans le client
Code Java (4 lignes)
AbstractLazyInitializer .setRemoteLazyLoadProxyCallback(new MeatClientLazyLoadProxyCallback()); AbstractPersistentCollection .setRemoteLazyLoadCollectionCallback(new MeatClientLazyLoadCollectionCallback());
Exemples d'implémentations des callbacks
Code Java (16 lignes)
public class MeatClientLazyLoadProxyCallback implements RemoteLazyLoadProxyCallback { // Thread.dumpStack(); id); if(result == null){ LogFactory.getLog(getClass()).error(entityName+" - "+ id +" is NULL ‹‹‹‹‹‹‹‹‹‹‹‹‹‹‹‹‹‹‹‹‹‹‹‹"); } return result; } }
Code Java (41 lignes)
public class MeatClientLazyLoadCollectionCallback implements RemoteLazyLoadCollectionCallback { public PersistentCollection lazyLoad(SessionImplementor session, PersistentCollection collection) { PersistentCollection result = ServiceLocator.getService( LazyInitFacade.class).initializeCollection(collection, true); Field field; if (collection instanceof PersistentSet) { field = ReflectionUtils.findField(PersistentSet.class, "set", } else if (collection instanceof PersistentBag) { field = ReflectionUtils.findField(PersistentBag.class, "bag", } else if (collection instanceof PersistentList) { field = ReflectionUtils.findField(PersistentList.class, "list", } else if (collection instanceof PersistentMap) { field = ReflectionUtils.findField(PersistentMap.class, "map", } else { .getClass() + " is not yet supported")); } "storedSnapshot"); if (snapshot != null) { ReflectionUtils.makeAccessible(snapshot); if (o == null) { } } ReflectionUtils.makeAccessible(field); ReflectionUtils.setField(field, collection, result); return result; } }

Source : indéterminée
Version en cache
01/07/2025 11:22:41 Cette version de la page est en cache (à la date du 01/07/2025 11:22:41) afin d'accélérer le traitement. Vous pouvez activer le mode utilisateur dans le menu en haut pour afficher la version plus récente de la page.Document créé le 13/09/2004, dernière modification le 26/10/2018
Source du document imprimé : https://www.gaudry.be/ast-rf-455.html
L'infobrol est un site personnel dont le contenu n'engage que moi. Le texte est mis à disposition sous licence CreativeCommons(BY-NC-SA). Plus d'info sur les conditions d'utilisation et sur l'auteur.